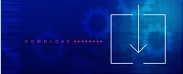

Since we want to use this provider as a wrapper, it should render any children it wraps. You can access this provider component using object dot notation ( newContext.Provider). The context you’ve previously created also contains a provider component your new provider will render. Here, you will use the context for the first time. The most important part is where the rendering happens, what follows the return statement. Again, this will make them available for components wrapped with the provider. You can also expose these functions by setting them as values for the provider.
REACT MULTIPLE CONTEXTS UPDATE
These functions can work with the state, update its values. You can also use useCallback hook to create memoized functions.
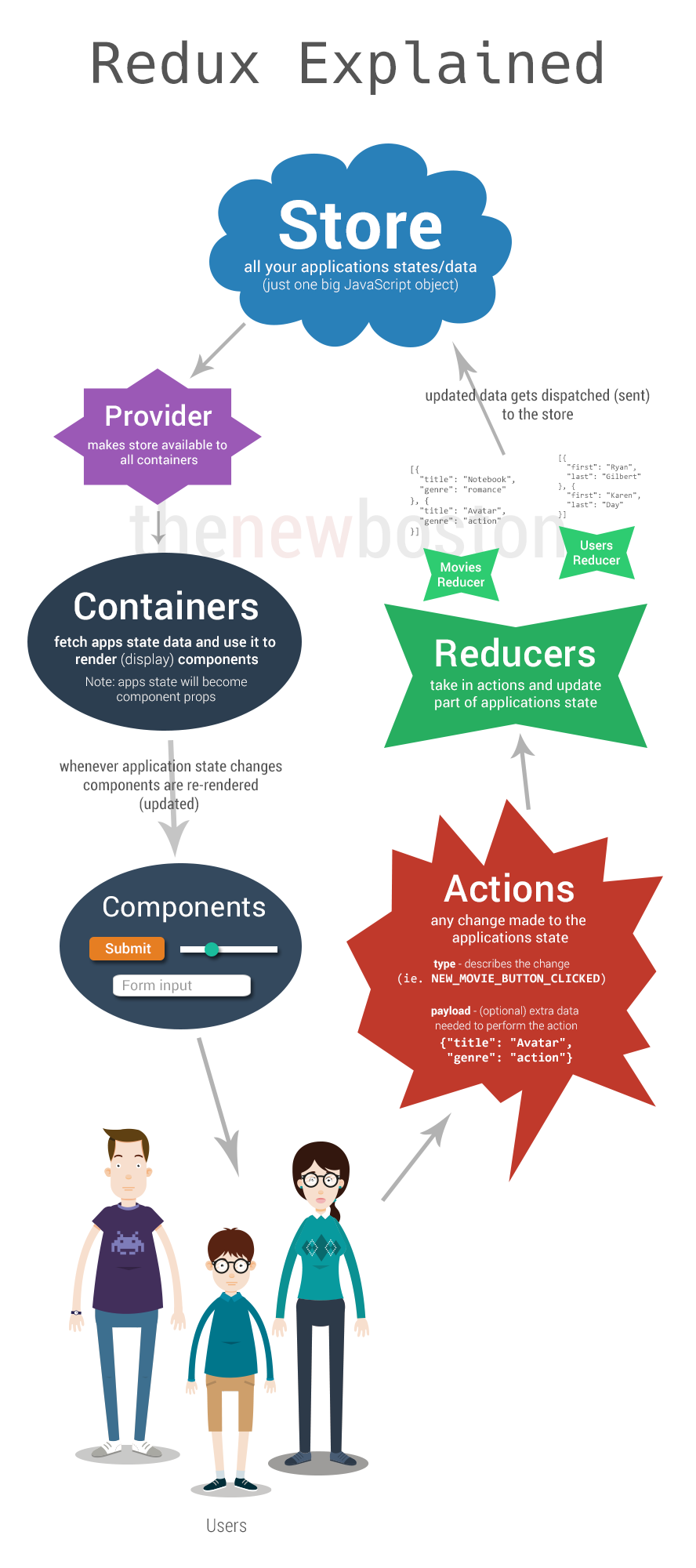
This will make it available for any component wrapped with the provider. You can then expose this state by setting it as a value for the provider. For example, you can use useState hook to create new state for the provider. Inside this component, you can use any react hook you want.
REACT MULTIPLE CONTEXTS CODE
It makes it easier to understand the code when you read it. It is a good practice to end the name with “Provider”. Nowadays, provider is usually created as a function component. Creating the context providerĬreating the provider is similar to creating a regular React component. This will ensure the app has access to all providers up the tree. If you have multiple providers, you can wrap one inside another while keeping the app as the last child. This guarantees that any component in the app will be able to communicate with the provider. In general, provider is used as a wrapper for the entire app. What matters is that the provider is used as a wrapper somewhere in the tree above. It doesn’t matter where in the component tree they are. This is important to remember.Ĭomponents will be able to communicate with provider only if they are provider’s children. Provider wraps all components that should be able to access the context. This provider is a component that provides your app with the value(s) stored inside the context. The second thing you have to do is to create a provider for your new context. This means we must export it from where it is.
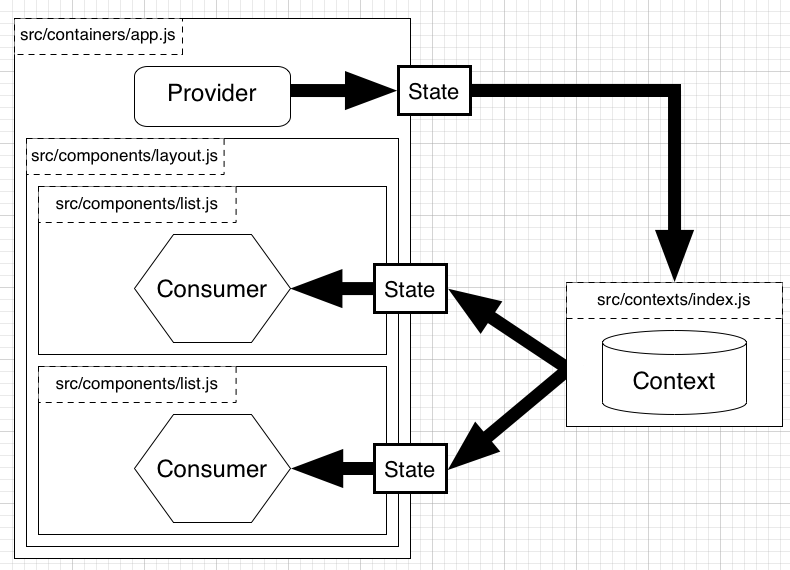
So, if we want to use the useContext hook to access the context anywhere in the app the context itself must be also accessible anywhere. The useContext hook accepts a context as a parameter. Also notice that we are exporting the context. Later, in the next step, you will add values to it. It will be empty just for now when you create it. This doesn’t mean this context will be empty forever. Notice that we are declaring the context as empty, basically assigning it undefined. Import createContext() method from React:Įxport const newContext = createContext() Well, at least one them because your React app can contain infinite number of contexts. This context will be the global state available for use across the app. You achieve this by using createContext() method shipped with React. Using React context requires getting done few things. This includes both, getting those values as well as changing them. Second, to work with values exposed through this context. First, to help you reach out to any context and from anywhere. The React useContext hook promises to help you with two things. This is the goal of the React useContext hook. Another solution is to skip all prop drilling and simply reach to the context from the component where you need those data. It would also go against the idea of having one source of truth that is globally accessible. However, this would lead to duplicate code. One way to solve this is making those data local. You may have to pass these data through multiple components. It can take a lot of prop drilling to get the data from the top to where you need them. They are often difficult to use in nested components. That said, there is one problem with these global states. React context API makes it easy to create these global states. Which one is the best choice depends on situation. You can have global states and you can have local states. Local only from the place where they are defined, and down the tree. When you work with data they are usually one of two types, global or local. React context, global states, prop drilling
REACT MULTIPLE CONTEXTS HOW TO
This tutorial will show you how to create new context, how to get values from it and how to change them. The useContext hook allows you to work with React contexts from anywhere and pass its data throughout your app. React context makes it easy to create globally accessible data, and states.
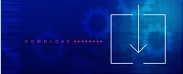